Factory Design Pattern
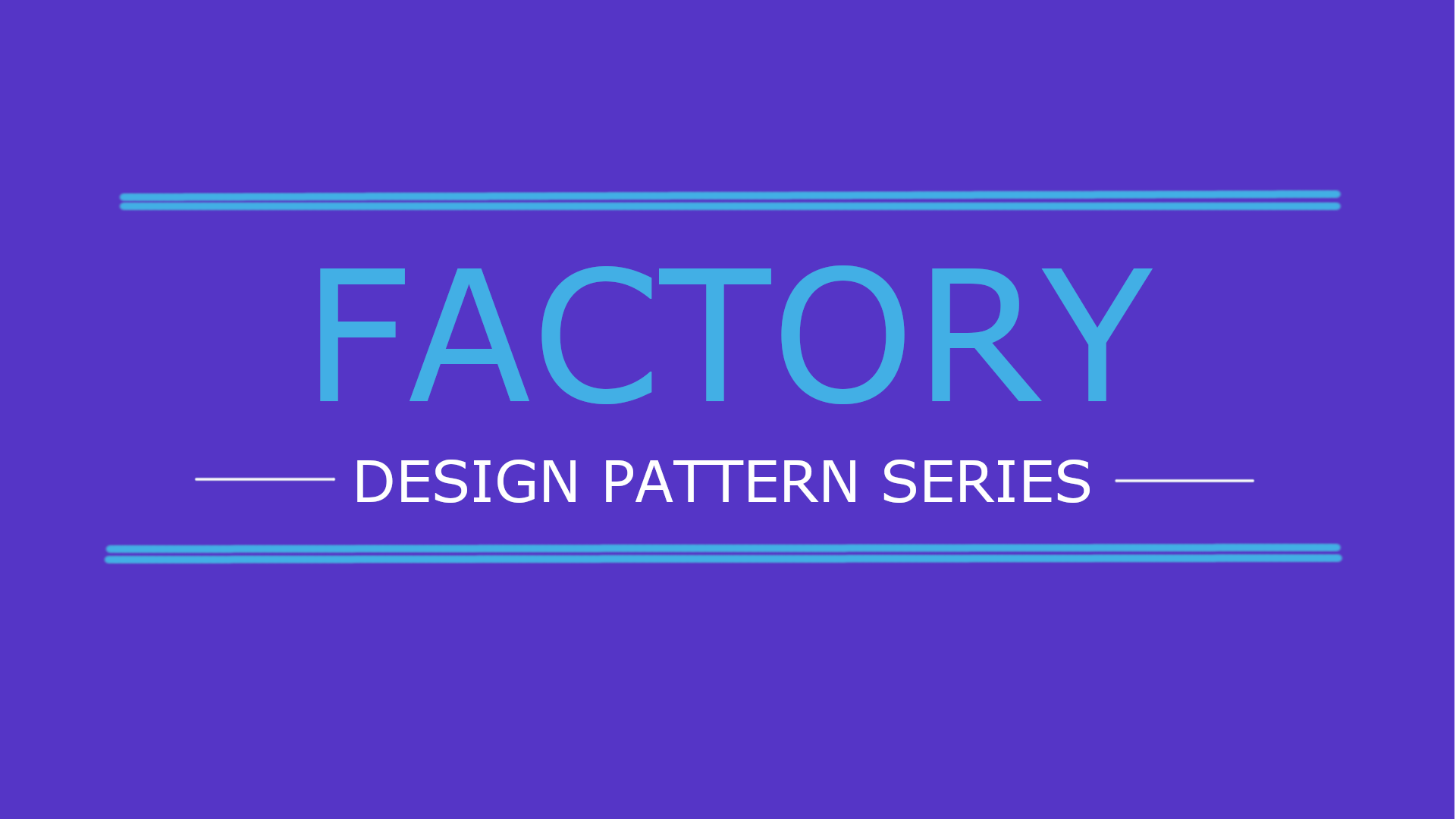
Factory design pattern is a creational pattern that uses factory methods to deal with the problem of creating objects without having to specify the exact class of the object that will be created.
In other words, factory design pattern is used when we have a super class with multiple sub-classes and based on input, we need to return one of the sub-class. This pattern takes the responsibility of instantiation of a class from client program to a factory class. Factory pattern is one of the most used design patterns in Java. This type of design pattern comes under creational pattern as this pattern provides one of the best ways to create an object.
/**
* This class is an abstract class.
*/
public abstract class Person {
public abstract String getFirstName();
public abstract String getLastName();
public abstract int getAge();
public abstract PersonType getType();
@Override
public String toString() {
return "[firstName=" + this.getFirstName() +
", lastName=" + this.getLastName() +
", age=" + this.getAge() +
", type=" + this.getType() +
"]";
}
}
/**
* This class is a sub-class of Person with values of Student hard coded.
*/
public class Student extends Person {
@Override
public String getFirstName() {
return "Sanchi";
}
@Override
public String getLastName() {
return "Goyal";
}
@Override
public int getAge() {
return 18;
}
@Override
public PersonType getType() {
return PersonType.STUDENT;
}
}
/**
* This class is a sub-class of Person with values of Teacher hard coded.
*/
public class Teacher extends Person {
@Override
public String getFirstName() {
return "Joe";
}
@Override
public String getLastName() {
return "Brown";
}
@Override
public int getAge() {
return 40;
}
@Override
public PersonType getType() {
return PersonType.TEACHER;
}
}
/**
* This class implements factory pattern. Its a facade that clients can use to obtain instances of
* type person.
*/
public class PersonFactory {
/**
* Returns the instance of Person based on the input type.
*/
public static Person getPerson(PersonType type) {
switch (type) {
case STUDENT:
return new Student();
case TEACHER:
return new Teacher();
default:
return null;
}
}
}
public enum PersonType {
STUDENT,
TEACHER
}
/**
* This is client code, which uses the factory to obtain instances of student and teacher.
*/
public class PersonClient {
public static void main(String[] args) {
Person student = PersonFactory.getPerson(PersonType.STUDENT);
System.out.println(student);
Person teacher = PersonFactory.getPerson(PersonType.TEACHER);
System.out.println(teacher);
}
}
Advantages of Factory Pattern
- Factory Design Pattern provides approach to code for interface rather than implementation.
- Factory design pattern removes the instantiation of actual implementation classes from client code. This simplifies writing unit tests for client code.
- Factory pattern provides abstraction.
Source code on Github